Java Not Within Range Try Again
This post is another addition in best practices series available in this blog. In this post, I am covering some well-known and some lilliputian known practices which you must consider while handling exceptions in your side by side java programming consignment. Follow this link to read more almost exception treatment in java.
Table of Contents Blazon of exceptions User defined custom exceptions All-time practices you must consider and follow Never swallow the exception in take hold of block Declare the specific checked exceptions that your method tin can throw Practice non take hold of the Exception course rather catch specific sub classes Never grab Throwable class Always correctly wrap the exceptions in custom exceptions then that stack trace is not lost Either log the exception or throw it merely never do the both Never throw any exception from finally block Always catch only those exceptions that y'all tin really handle Don't utilise printStackTrace() statement or similar methods Employ finally blocks instead of catch blocks if you are not going to handle exception Remember "Throw early grab late" principle Ever clean up after treatment the exception Throw only relevant exception from a method Never employ exceptions for flow command in your program Validate user input to catch agin weather condition very early in request processing Ever include all information nearly an exception in single log message Pass all relevant information to exceptions to make them informative every bit much as possible Always terminate the thread which it is interrupted Employ template methods for repeated try-grab Document all exceptions in your application in javadoc
Before nosotros swoop into deep concepts of exception handling best practices, lets showtime with i of the most important concepts which is to understand that at that place are three general types of throwable classes in Coffee: checked exceptions, unchecked exceptions, and errors.
1. Blazon of exceptions in Java
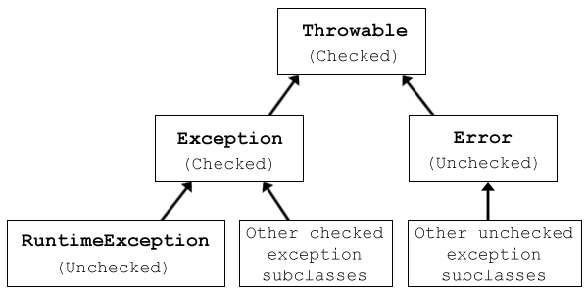
Checked exceptions
These are exceptions that must exist declared in the throws clause of a method. They extend Exception and are intended to be an "in your face" type of exceptions. Java wants you to handle them considering they somehow are dependent on external factors outside your programme. A checked exception indicates an expected problem that can occur during normal system operation. Mostly these exception happen when you try to use external systems over network or in file organisation. By and large, the correct response to a checked exception should be to endeavor again later, or to prompt the user to modify his input.
Unchecked exceptions
These are exceptions that do not need to be declared in a throws clause. JVM merely doesn't force you lot to handle them as they are by and large generated at runtime due to programmatic errors. They extend RuntimeException. The most common example is a NullPointerException
[Quite scary.. Isn't it?]. An unchecked exception probably shouldn't be retried, and the correct action should be usually to do nothing, and permit it come up out of your method and through the execution stack. At a high level of execution, this blazon of exceptions should be logged.
Errors
are serious runtime environment problems that are almost certainly not recoverable. Some examples are OutOfMemoryError, LinkageError, and StackOverflowError. They mostly crash y'all program or role of program. Simply a skillful logging do will help you in determining the exact causes of errors.
two. User defined custom exceptions
Anytime when user feels that he wants to use its ain application specific exception for some reasons, he can create a new grade extending appropriate super grade (mostly its Exception
) and offset using it in appropriate places. These user defined exceptions can be used in two means:
- Either directly throw the custom exception when something goes wrong in application
throw new DaoObjectNotFoundException("Couldn't find dao with id " + id);
- Or wrap the original exception within custom exception and throw information technology
catch (NoSuchMethodException east) { throw new DaoObjectNotFoundException("Couldn't find dao with id " + id, due east); }
Wrapping an exception can provide extra information to the user past calculation your own message/ context data, while still preserving the stack trace and message of the original exception. It also allows you lot to hide the implementation details of your code, which is the virtually important reason to wrap exceptions.
Now lets start exploring the best practices followed for exception treatment industry wise.
3. Coffee exception handling all-time practices y'all must consider and follow
3.1. Never consume the exception in catch block
grab (NoSuchMethodException east) { return aught; }
Doing this not merely return "null" instead of treatment or re-throwing the exception, information technology totally swallows the exception, losing the cause of error forever. And when you don't know the reason of failure, how you would foreclose it in time to come? Never practise this !!
3.2. Declare the specific checked exceptions that your method tin can throw
public void foo() throws Exception { //Incorrect way }
Always avoid doing this as in in a higher place lawmaking sample. It simply defeats the whole purpose of having checked exception. Declare the specific checked exceptions that your method can throw. If there are just too many such checked exceptions, y'all should probably wrap them in your own exception and add data to in exception bulletin. Yous tin also consider lawmaking refactoring likewise if possible.
public void foo() throws SpecificException1, SpecificException2 { //Correct way }
iii.three. Exercise not take hold of the Exception class rather catch specific sub classes
try { someMethod(); } grab (Exception e) { LOGGER.mistake("method has failed", e); }
The problem with catching Exception is that if the method you are calling after adds a new checked exception to its method signature, the developer's intent is that you should handle the specific new exception. If your code simply catches Exception (or Throwable), you'll never know nigh the modify and the fact that your code is now incorrect and might break at any betoken of time in runtime.
3.four. Never catch Throwable grade
Well, its one pace more serious problem. Because java errors are also subclasses of the Throwable. Errors are irreversible conditions that tin not be handled by JVM itself. And for some JVM implementations, JVM might non actually even invoke your catch clause on an Error.
3.5. Always correctly wrap the exceptions in custom exceptions so that stack trace is not lost
catch (NoSuchMethodException e) { throw new MyServiceException("Some information: " + due east.getMessage()); //Incorrect fashion }
This destroys the stack trace of the original exception, and is always wrong. The correct style of doing this is:
catch (NoSuchMethodException eastward) { throw new MyServiceException("Some information: " , e); //Correct way }
three.6. Either log the exception or throw it merely never do the both
catch (NoSuchMethodException e) { LOGGER.fault("Some information", e); throw e; }
As in above example lawmaking, logging and throwing will result in multiple log messages in log files, for a unmarried problem in the code, and makes life hell for the engineer who is trying to dig through the logs.
iii.7. Never throw any exception from finally block
try { someMethod(); //Throws exceptionOne } finally { cleanUp(); //If finally as well threw any exception the exceptionOne volition be lost forever }
This is fine, as long equally cleanUp() tin can never throw any exception. In the above example, if someMethod() throws an exception, and in the finally block also, cleanUp() throws an exception, that 2d exception will come out of method and the original first exception (correct reason) will be lost forever. If the code that you lot telephone call in a finally block can possibly throw an exception, make sure that you either handle it, or log information technology. Never let it come out of the finally block.
3.viii. Always catch just those exceptions that y'all can actually handle
catch (NoSuchMethodException e) { throw e; //Avoid this as it doesn't assistance anything }
Well this is most of import concept. Don't catch any exception just for the sake of catching it. Take hold of any exception but if you lot want to handle information technology or, y'all want to provide additional contextual information in that exception. If you can't handle information technology in catch cake, and so best communication is just don't catch it simply to re-throw it.
three.ix. Don't utilise printStackTrace() statement or like methods
Never leave printStackTrace() after finishing your code. Chances are ane of your young man colleague will go one of those stack traces eventually, and have exactly cipher knowledge as to what to exercise with it considering it volition non have whatsoever contextual information appended to information technology.
3.10. Utilize finally blocks instead of catch blocks if y'all are not going to handle exception
try { someMethod(); //Method two } finally { cleanUp(); //do cleanup hither }
This is too a skillful do. If within your method yous are accessing some method 2, and method 2 throw some exception which you exercise not want to handle in method 1, just even so want some cleanup in case exception occur, then do this cleanup in finally block. Do not use grab cake.
iii.11. Remember "Throw early grab late" principle
This is probably the about famous principle about Exception handling. Information technology basically says that you should throw an exception as soon equally you lot can, and take hold of it belatedly as much every bit possible. You should wait until you take all the information to handle it properly.
This principle implicitly says that you volition be more likely to throw it in the low-level methods, where you will be checking if single values are null or not appropriate. And yous will be making the exception climb the stack trace for quite several levels until you attain a sufficient level of abstraction to be able to handle the problem.
3.12. Always clean up later handling the exception
If you lot are using resources like database connections or network connections, make certain you clean them upward. If the API you are invoking uses merely unchecked exceptions, you should however clean up resources after use, with attempt – finally blocks. Within try block admission the resource and inside finally shut the resource. Even if any exception occur in accessing the resource, then also resource volition be closed gracefully.
3.13. Throw only relevant exception from a method
Relevancy is important to proceed application clean. A method which tries to read a file; if throws NullPointerException then it will non give any relevant data to user. Instead it will exist better if such exception is wrapped within custom exception due east.g. NoSuchFileFoundException then information technology volition be more useful for users of that method.
3.14. Never use exceptions for menstruum control in your program
We take read it many times but sometimes we keep seeing lawmaking in our project where developer tries to use exceptions for awarding logic. Never do that. It makes code hard to read, empathize and ugly.
iii.xv. Validate user input to grab adverse conditions very early in asking processing
Ever validate user input in very early on stage, fifty-fifty earlier it reached to actual controller. Information technology will help you to minimize the exception handling code in your core application logic. It besides helps y'all in making awarding consistent if there is some error in user input.
For case: If in user registration awarding, y'all are post-obit below logic:
ane) Validate User
2) Insert User
3) Validate address
4) Insert accost
5) If problem the Rollback everything
This is very incorrect approach. Information technology tin can exit you database in inconsistent state in diverse scenarios. Rather validate everything in first place and and so accept the user information in dao layer and make DB updates. Correct approach is:
ane) Validate User
2) Validate accost
iii) Insert User
iv) Insert address
5) If problem the Rollback everything
iii.16. Ever include all information about an exception in single log bulletin
LOGGER.debug("Using enshroud sector A"); LOGGER.debug("Using retry sector B");
Don't do this.
Using a multi-line log message with multiple calls to LOGGER.debug() may look fine in your examination case, but when information technology shows upwards in the log file of an app server with 400 threads running in parallel, all dumping data to the same log file, your two log messages may end up spaced out 1000 lines apart in the log file, fifty-fifty though they occur on subsequent lines in your code.
Practice it similar this:
LOGGER.debug("Using cache sector A, using retry sector B");
3.17. Pass all relevant information to exceptions to make them informative as much as possible
This is too very important to brand exception messages and stack traces useful and informative. What is the use of a log, if you are non able to determine annihilation out of it. These type of logs just exist in your lawmaking for ornament purpose.
3.eighteen. Ever terminate the thread which it is interrupted
while (true) { try { Thread.sleep(100000); } catch (InterruptedException due east) {} //Don't exercise this doSomethingCool(); }
InterruptedException is a clue to your code that it should end whatever it'southward doing. Some common use cases for a thread getting interrupted are the active transaction timing out, or a thread pool getting close down. Instead of ignoring the InterruptedException, your code should practise its best to finish up what it's doing, and finish the current thread of execution. And then to correct the example above:
while (truthful) { effort { Thread.sleep(100000); } catch (InterruptedException e) { break; } } doSomethingCool();
3.19. Use template methods for repeated attempt-catch
At that place is no employ of having a like catch block in 100 places in your code. It increases code duplicity which does not assist anything. Use template methods for such cases.
For example below lawmaking tries to shut a database connection.
class DBUtil{ public static void closeConnection(Connection conn){ try{ conn.close(); } catch(Exception ex){ //Log Exception - Cannot close connection } } }
This type of method volition be used in thousands of places in your application. Don't put whole code in every place rather ascertain above method and use information technology everywhere like below:
public void dataAccessCode() { Connection conn = naught; attempt{ conn = getConnection(); .... } finally{ DBUtil.closeConnection(conn); } }
3.20. Document all exceptions in the application with javadoc
Make information technology a do to javadoc all exceptions which a piece of lawmaking may throw at runtime. Besides try to include possible class of action, user should follow in example these exception occur.
That's all i take in my mind for now related to Java exception handling best practices. If you found anything missing or you lot does not relate to my view on any point, driblet me a comment. I will be happy to discuss.
Happy Learning !!
Source: https://howtodoinjava.com/best-practices/java-exception-handling-best-practices/
0 Response to "Java Not Within Range Try Again"
Post a Comment